vue3+vite自定义指令文件,统一管理指令文件
Sonder
2023-12-20
2465字
6分钟
浏览 (2.4k)
指令文件
/src/directive/index.js
export default {
install: function (app) {
const directives = import.meta.glob('./**/index.js', { eager: true });
for (const key in directives) {
if (key === './index.js') return;
const directive = directives[key];
const name = key.replace(/\.\/|\/index.js/g, '');
app.directive(name, directive.default || directive);
}
}
};
添加到main.js里面
// 自定义指令
import Directive from '@/directive';
const app = createApp(App);
app.use(Directive).use(router).mount('#app');
例如防抖指令
\src\directive\debounce\index.js
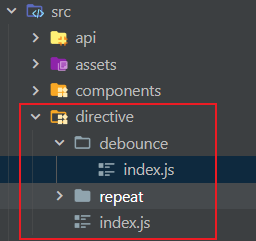
/**
* 按钮防抖
* 用法:<el-button v-debounce="submit">点击</el-button>
*/
export default {
mounted(el, binding) {
if (typeof binding.value !== 'function') {
console.error('callback must be a function')
return;
}
let timer = null
el.__handleClick__ = function () {
if (timer) {
clearInterval(timer)
}
timer = setTimeout(() => {
binding.value()
}, 200)
}
el.addEventListener('click', el.__handleClick__)
},
beforeUnmount(el) {
el.removeEventListener('click', el.__handleClick__)
}
};
nuxt3如何创建指令呢
在 plugins 里面新建 directive.client.js
,统一所有指令
src\plugins\directive.client.js
const modulesFiles = import.meta.glob('../utils/directives/*.js', { eager: true })
const directives = {}
for (const path in modulesFiles) {
const moduleName = path.replace(/.*\/([^/]+)\.js$/, '$1')
directives[moduleName] = modulesFiles[path].default
}
// 定义nuxt插件
export default defineNuxtPlugin((nuxtApp) => {
// 通过循环注册所有指令
for (const key in directives)
nuxtApp.vueApp.directive(key, directives[key])
})
创建指令
src\utils\directives\money.js
/**
* 文本展示为金额:20 -> 20.00
*/
export default {
mounted(el) {
let text = el.textContent.trim()
const hasTag = text.includes('¥')
// 检查文本内容是否包含 '¥' 符号,如果包含则移除
if (hasTag)
text = text.replace('¥', '')
// 检查文本内容是否是数字或可以转换为数字的字符串
if (!isNaN(text)) {
// 将文本内容转换为数字并四舍五入保留两位小数
const roundedNumber = Number(text).toFixed(2)
// 更新元素的文本内容为四舍五入后的值
el.textContent = hasTag ? `¥${roundedNumber}` : roundedNumber
}
}
}