js 任务队列函数
Sonder
2024-01-24
1984字
5分钟
浏览 (2.7k)
/**
* 表示一个函数队列, 按照指定的间隔顺序执行函数。
*/
export class FunctionQueue {
/**
* 创建 FunctionQueue 的实例。
* @param {number} [interval] - 函数执行的间隔时间(毫秒)。默认为 500 毫秒。
*/
constructor(interval = 500) {
/**
* 函数队列, 存储待执行的函数。
* @type {Function[]}
*/
this.queue = []
/**
* setInterval 函数的计时器标识。
* @type {number|null}
*/
this.timer = null
/**
* 函数执行的间隔时间(毫秒)。
* @type {number}
*/
this.interval = interval
/**
* 标识是否正在执行函数。
* @type {boolean}
*/
this.isExecuting = false
}
/**
* 将函数添加到队列中, 并立即触发执行。
* @param {Function} callback - 要添加到队列中的函数。
*/
addToQueue(callback) {
this.queue.push(callback)
if (!this.isExecuting)
this.executeNext()
}
/**
* 执行队列中的下一个函数。
* @private
*/
async executeNext() {
if (this.isExecuting || this.queue.length === 0)
return
this.isExecuting = true
const callback = this.queue.shift()
try {
await callback()
}
catch (error) {
console.error('执行函数时发生错误:', error)
}
this.isExecuting = false
if (this.queue.length > 0) {
setTimeout(() => {
this.executeNext()
}, this.interval)
}
}
}
使用:
import { FunctionQueue } from '@/utils'
const pushMessageQueue = new FunctionQueue(3000) // 3000ms内只执行一次
const messageList = [
{id:1, name: '消息1'},
{id:2, name: '消息2'},
{id:3, name: '消息3'},
{id:4, name: '消息4'},
{id:5, name: '消息5'},
]
messageList.forEach((message) => {
pushMessageQueue.addToQueue(() => {
messageListVal.push(message)
console.log(`=====>messageListVal`,messageListVal)
})
})
这样每三秒push一条数据了
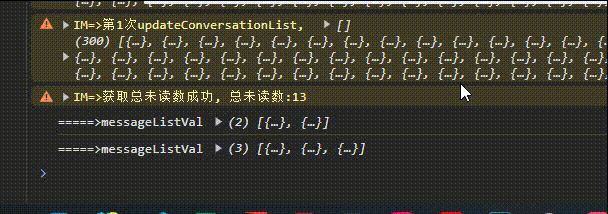